How Builders Can Improve Their Debugging Competencies By Gustavo Woltmann
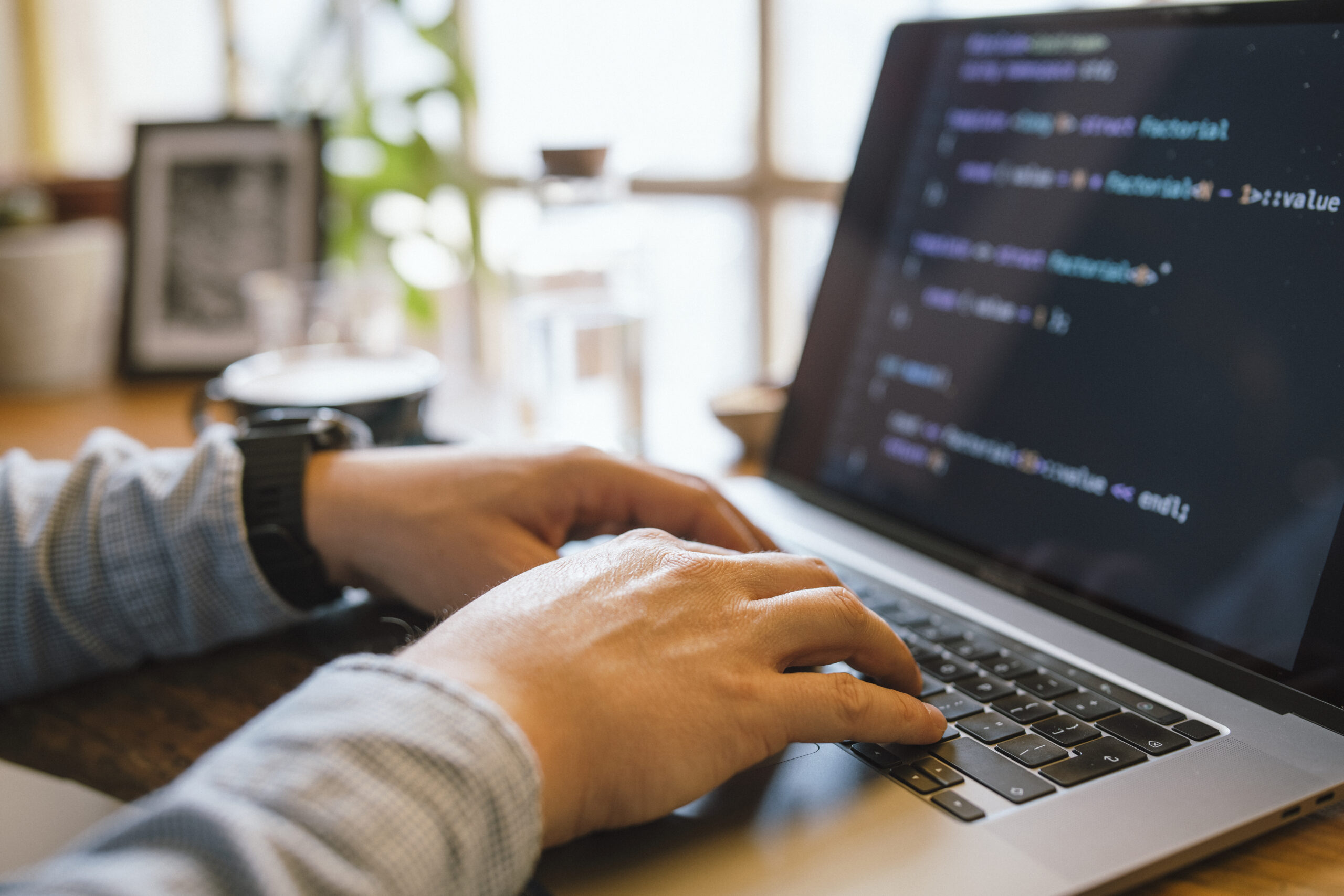
Debugging is The most necessary — yet frequently neglected — techniques inside of a developer’s toolkit. It isn't really just about fixing broken code; it’s about comprehension how and why points go Completely wrong, and Studying to Feel methodically to resolve troubles successfully. Whether you're a rookie or a seasoned developer, sharpening your debugging abilities can conserve hrs of stress and considerably transform your productiveness. Allow me to share many procedures that will help builders amount up their debugging video game by me, Gustavo Woltmann.
Learn Your Instruments
Among the list of quickest techniques developers can elevate their debugging competencies is by mastering the instruments they use every single day. Although creating code is one Element of progress, being aware of the best way to interact with it correctly for the duration of execution is equally vital. Modern-day growth environments come Geared up with effective debugging capabilities — but many builders only scratch the surface of what these resources can perform.
Just take, as an example, an Built-in Growth Atmosphere (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These equipment allow you to established breakpoints, inspect the worth of variables at runtime, phase through code line by line, and in many cases modify code within the fly. When made use of accurately, they let you observe accurately how your code behaves for the duration of execution, which is priceless for monitoring down elusive bugs.
Browser developer equipment, for example Chrome DevTools, are indispensable for front-finish builders. They permit you to inspect the DOM, watch network requests, look at real-time functionality metrics, and debug JavaScript in the browser. Mastering the console, sources, and community tabs can turn annoying UI challenges into manageable duties.
For backend or procedure-level builders, tools like GDB (GNU Debugger), Valgrind, or LLDB present deep Handle over managing procedures and memory management. Discovering these resources might have a steeper Discovering curve but pays off when debugging functionality troubles, memory leaks, or segmentation faults.
Over and above your IDE or debugger, grow to be snug with Model Manage units like Git to comprehend code record, uncover the exact instant bugs were launched, and isolate problematic improvements.
Ultimately, mastering your resources usually means likely further than default settings and shortcuts — it’s about producing an personal understanding of your enhancement surroundings to make sure that when problems occur, you’re not lost in the dead of night. The higher you are aware of your resources, the more time you may expend solving the actual dilemma rather then fumbling by means of the method.
Reproduce the condition
Probably the most crucial — and often overlooked — steps in effective debugging is reproducing the problem. Before leaping into your code or building guesses, developers want to make a steady surroundings or situation exactly where the bug reliably seems. With out reproducibility, fixing a bug results in being a video game of possibility, usually leading to squandered time and fragile code variations.
Step one in reproducing an issue is accumulating just as much context as you possibly can. Talk to issues like: What actions triggered The problem? Which environment was it in — progress, staging, or creation? Are there any logs, screenshots, or mistake messages? The greater element you might have, the less difficult it gets to be to isolate the precise circumstances below which the bug takes place.
As soon as you’ve gathered ample information, endeavor to recreate the issue in your neighborhood atmosphere. This might mean inputting the exact same information, simulating very similar user interactions, or mimicking technique states. If the issue appears intermittently, take into consideration creating automatic tests that replicate the edge scenarios or state transitions concerned. These assessments not only support expose the problem but in addition reduce regressions Later on.
Often, The difficulty might be setting-unique — it might take place only on selected functioning methods, browsers, or beneath unique configurations. Using resources like virtual devices, containerization (e.g., Docker), or cross-browser tests platforms is usually instrumental in replicating this kind of bugs.
Reproducing the challenge isn’t merely a move — it’s a mindset. It demands persistence, observation, plus a methodical tactic. But when you finally can consistently recreate the bug, you're presently halfway to repairing it. Which has a reproducible state of affairs, You should use your debugging resources a lot more proficiently, take a look at opportunity fixes properly, and connect extra Evidently with all your workforce or buyers. It turns an summary grievance into a concrete obstacle — Which’s the place developers thrive.
Study and Comprehend the Error Messages
Mistake messages will often be the most beneficial clues a developer has when a thing goes Completely wrong. Rather then observing them as annoying interruptions, developers ought to learn to take care of mistake messages as direct communications in the system. They normally inform you just what happened, where by it took place, and at times even why it happened — if you know the way to interpret them.
Start out by looking through the message diligently and in complete. Lots of builders, especially when underneath time strain, glance at the 1st line and quickly start making assumptions. But further within the mistake stack or logs could lie the legitimate root lead to. Don’t just copy and paste mistake messages into search engines like yahoo — read and fully grasp them initial.
Crack the error down into sections. Is it a syntax mistake, a runtime exception, or a logic mistake? Will it level to a certain file and line quantity? What module or purpose triggered it? These issues can manual your investigation and position you toward the accountable code.
It’s also practical to comprehend the terminology of your programming language or framework you’re making use of. Mistake messages in languages like Python, JavaScript, or Java often observe predictable patterns, and Understanding to acknowledge these can significantly accelerate your debugging method.
Some faults are vague or generic, and in All those cases, it’s vital to look at the context wherein the error transpired. Check out similar log entries, input values, and recent alterations during the codebase.
Don’t overlook compiler or linter warnings both. These typically precede larger sized issues and provide hints about prospective bugs.
In the long run, mistake messages are usually not your enemies—they’re your guides. Studying to interpret them appropriately turns chaos into clarity, supporting you pinpoint difficulties faster, decrease debugging time, and become a a lot more productive and self-confident developer.
Use Logging Correctly
Logging is One of the more impressive tools in a developer’s debugging toolkit. When utilized successfully, it provides genuine-time insights into how an application behaves, assisting you comprehend what’s taking place under the hood without needing to pause execution or step through the code line by line.
A good logging strategy starts off with recognizing what to log and at what amount. Prevalent logging degrees include things like DEBUG, Details, WARN, ERROR, and FATAL. Use DEBUG for detailed diagnostic information during enhancement, Facts for typical gatherings (like profitable start off-ups), WARN for potential challenges that don’t split the appliance, ERROR for genuine troubles, and FATAL when the procedure can’t keep on.
Stay away from flooding your logs with excessive or irrelevant details. An excessive amount logging can obscure critical messages and slow down your procedure. Target important events, state improvements, input/output values, and important determination points in the code.
Format your log messages Evidently and persistently. Consist of context, which include timestamps, request IDs, and performance names, so it’s simpler to trace issues in dispersed systems or multi-threaded environments. Structured logging (e.g., JSON logs) may make it even easier to parse and filter logs programmatically.
Through debugging, logs Allow you to keep track of how variables evolve, what circumstances are achieved, and what branches of logic are executed—all without having halting This system. They’re Particularly precious in manufacturing environments wherever stepping via code isn’t doable.
In addition, use logging frameworks and instruments (like Log4j, Winston, or Python’s logging module) that support log rotation, filtering, and integration with monitoring dashboards.
Finally, sensible logging is about harmony and clarity. Which has a effectively-considered-out logging approach, it is possible to lessen the time it will take to spot difficulties, acquire deeper visibility into your apps, and Increase the General maintainability and dependability of your respective code.
Feel Similar to a Detective
Debugging is not just a specialized endeavor—it's a type of investigation. To proficiently identify and repair bugs, developers have to solution the process like a detective solving a thriller. This frame of mind can help stop working complex problems into manageable elements and comply with clues logically to uncover the basis bring about.
Get started by accumulating proof. Look at the symptoms of the issue: error messages, incorrect output, or efficiency concerns. Similar to a detective surveys a criminal offense scene, acquire as much appropriate data as it is possible to devoid of leaping to conclusions. Use logs, take a look at scenarios, and consumer studies to piece collectively a clear image of what’s occurring.
Following, kind hypotheses. Request oneself: What could possibly be leading to this behavior? Have any changes recently been built to your codebase? Has this challenge transpired prior to under identical situation? The purpose is always to narrow down alternatives and establish likely culprits.
Then, check your theories systematically. Attempt to recreate the condition in a very controlled environment. For those who suspect a certain perform or ingredient, isolate it and confirm if the issue persists. Just like a detective conducting interviews, request your code concerns and Enable the final results lead you nearer to the truth.
Pay near interest to compact information. Bugs frequently disguise inside the the very least anticipated places—just like a missing semicolon, an off-by-just one error, or simply a race problem. Be complete and individual, resisting the urge to patch The difficulty devoid of totally knowledge it. Short-term fixes may well hide the true problem, only for it to resurface afterwards.
Finally, retain notes on Everything you tried out and learned. Just as detectives log their investigations, documenting your debugging system can conserve time for long run issues and support others recognize your reasoning.
By wondering like a detective, developers can sharpen their analytical techniques, approach difficulties methodically, and develop into more practical at uncovering hidden concerns in advanced more info units.
Create Exams
Producing checks is among the most effective methods to increase your debugging techniques and overall improvement effectiveness. Exams not merely enable capture bugs early but will also function a security Web that gives you self confidence when building variations to your codebase. A well-tested application is easier to debug because it allows you to pinpoint precisely exactly where and when an issue occurs.
Start with unit tests, which concentrate on personal functions or modules. These little, isolated tests can rapidly reveal whether a specific bit of logic is Performing as predicted. Each time a examination fails, you right away know in which to appear, considerably reducing some time expended debugging. Unit exams are especially useful for catching regression bugs—challenges that reappear immediately after Earlier getting fixed.
Future, combine integration exams and end-to-close assessments into your workflow. These aid make sure that many portions of your application do the job jointly easily. They’re particularly handy for catching bugs that take place in complicated devices with a number of components or expert services interacting. If a thing breaks, your exams can show you which Portion of the pipeline unsuccessful and beneath what conditions.
Producing tests also forces you to definitely Believe critically regarding your code. To test a aspect effectively, you would like to grasp its inputs, expected outputs, and edge situations. This level of comprehension naturally sales opportunities to better code construction and much less bugs.
When debugging an issue, composing a failing exam that reproduces the bug could be a robust first step. After the take a look at fails regularly, it is possible to focus on fixing the bug and enjoy your test pass when The problem is fixed. This approach makes sure that the exact same bug doesn’t return in the future.
In brief, producing checks turns debugging from a irritating guessing match right into a structured and predictable process—assisting you catch far more bugs, faster and even more reliably.
Just take Breaks
When debugging a tough difficulty, it’s easy to become immersed in the trouble—watching your display screen for several hours, hoping solution soon after Option. But One of the more underrated debugging tools is simply stepping away. Taking breaks helps you reset your mind, decrease disappointment, and often see the issue from a new perspective.
When you're too close to the code for too long, cognitive exhaustion sets in. You might start overlooking noticeable faults or misreading code that you choose to wrote just several hours before. With this condition, your brain gets to be much less efficient at problem-resolving. A brief stroll, a coffee crack, or maybe switching to a unique process for 10–quarter-hour can refresh your aim. Quite a few developers report discovering the root of a problem when they've taken time for you to disconnect, letting their subconscious work during the qualifications.
Breaks also aid prevent burnout, Primarily through more time debugging sessions. Sitting down in front of a monitor, mentally caught, is not just unproductive but will also draining. Stepping absent enables you to return with renewed Electrical power and also a clearer attitude. You might quickly recognize a lacking semicolon, a logic flaw, or possibly a misplaced variable that eluded you prior to.
When you’re stuck, a very good guideline should be to set a timer—debug actively for forty five–60 minutes, then have a 5–10 moment break. Use that point to move all around, stretch, or do anything unrelated to code. It may come to feel counterintuitive, especially beneath limited deadlines, nevertheless it basically contributes to a lot quicker and simpler debugging In the long term.
In brief, getting breaks is not a sign of weak point—it’s a sensible method. It offers your Mind Area to breathe, increases your perspective, and aids you steer clear of the tunnel eyesight that often blocks your progress. Debugging is often a psychological puzzle, and rest is a component of fixing it.
Master From Every Bug
Every single bug you come upon is more than just A brief setback—It can be a possibility to grow like a developer. No matter if it’s a syntax mistake, a logic flaw, or a deep architectural problem, each can train you a thing important in the event you take some time to mirror and assess what went Erroneous.
Get started by inquiring yourself a couple of crucial inquiries when the bug is solved: What brought about it? Why did it go unnoticed? Could it are caught before with improved tactics like device tests, code opinions, or logging? The responses generally reveal blind places in your workflow or understanding and help you build stronger coding habits going ahead.
Documenting bugs can even be an outstanding practice. Hold a developer journal or sustain a log where you note down bugs you’ve encountered, the way you solved them, and Anything you figured out. After some time, you’ll start to see designs—recurring problems or common mistakes—you could proactively prevent.
In crew environments, sharing Everything you've discovered from the bug using your peers can be Primarily strong. Regardless of whether it’s through a Slack information, a short write-up, or A fast information-sharing session, assisting Many others stay away from the exact same difficulty boosts crew efficiency and cultivates a more robust Understanding society.
Far more importantly, viewing bugs as lessons shifts your way of thinking from disappointment to curiosity. In lieu of dreading bugs, you’ll start off appreciating them as important portions of your advancement journey. In fact, several of the best developers are not the ones who generate excellent code, but individuals that constantly master from their errors.
Eventually, Every bug you deal with adds a fresh layer towards your skill set. So future time you squash a bug, take a second to replicate—you’ll come away a smarter, far more able developer due to it.
Summary
Improving upon your debugging abilities normally takes time, observe, and patience — even so the payoff is large. It makes you a more productive, self-assured, and able developer. The next time you are knee-deep in a very mysterious bug, try to remember: debugging isn’t a chore — it’s an opportunity to become superior at Anything you do.